Mastering Python Programming: A Step-by-Step Guide for Beginners
- primaraldinternshi
- Apr 1
- 3 min read
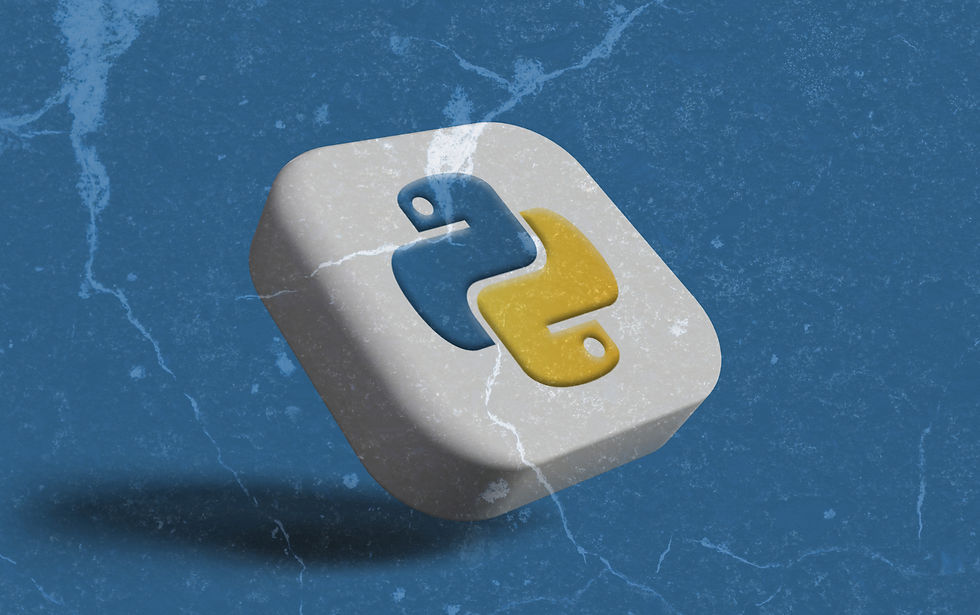
Funnily enough, I remember when someone once asked me, "What does Python mean in programming? Is it like a snake or what?" I laughed and told them, "Well, it’s not a snake, but it’s just as powerful and adaptable!" Python was actually named after the British comedy series Monty Python’s Flying Circus, not the reptile. But despite its quirky name, it’s one of the most beginner-friendly yet powerful programming languages in the world.
Whether you dream of building websites, diving into data science, or automating mundane tasks, Python has got you covered. But let’s be honest, starting out can feel like learning a new language (literally!). You might be asking: Where do I begin? How do I stay consistent? Well, this guide is here to walk you through Python step by step, making sure you not only understand but enjoy the process.
Step 1: Setting Up Your Python Environment - Mastering Python Programming
Before you start coding, you need to install Python. Visit python.org to download the latest version. During installation, make sure to check the box that says “Add Python to PATH” to avoid headaches later.
To verify your installation, open your terminal or command prompt and type:
python --version
If it prints something like Python 3.x.x, congrats! You’re all set. Now, let’s start coding.
Choosing a Code Editor
While you can write Python code in any text editor, using a code editor or an Integrated Development Environment (IDE) will make your life easier. Popular options include:
Visual Studio Code (VS Code):- Lightweight and packed with useful extensions.
PyCharm:- Great for large projects, offering powerful debugging features.
Jupyter Notebook:- Ideal for data science and interactive coding.
Sublime Text:- Fast and simple with excellent Python support.
Download and install your preferred editor to start coding comfortably.
![]() | ![]() | ![]() | ![]() |
Step 2: Understanding Python Basics
Python’s syntax is simple and easy to read. Let’s begin with the traditional “Hello, World!” program:
print("Hello, World!")
Run this in your Python environment, and if you see Hello, World!, you’ve officially written your first Python program!
Variables and Data Types
Python doesn’t require you to declare variables explicitly. You can simply assign values like this:
name = "Alice"
age = 25
is_student = True
The cool thing? You don’t need to specify data types, Python does it for you!
User Input
Want your program to interact with users? Try this:
name = input("What is your name? ")
print("Nice to meet you, " + name + "!")
Go ahead, run it, and have a conversation with your Python script.
Step 3: Control Flow - Making Decisions
Programs often need to make decisions based on conditions. Here’s a simple if-else statement:
age = int(input("Enter your age: "))
if age >= 18:
print("You're an adult!")
else:
print("You're still a minor!")
Python uses indentation (not curly braces) to define code blocks, making it super readable.
Step 4: Loops - Automating Repetitive Tasks
Loops help you repeat actions without writing the same code multiple times.
The for Loop
for i in range(5):
print("Iteration", i)
The while Loop
count = 0
while count < 5:
print("Count is", count)
count += 1
Try modifying these loops to understand them better.
Step 5: Functions - Reusability at Its Best
Functions help break your code into reusable chunks. Here’s a simple example:
def greet(name):
return "Hello, " + name + "!"
print(greet("Alice"))
Functions keep your code neat and organized. The more you use them, the more efficient your programs become.
Step 6: Lists and Dictionaries - Storing and Organizing Data
Lists are used to store multiple values:
fruits = ["Apple", "Banana", "Cherry"]
print(fruits[0]) # Output: Apple
Dictionaries use key-value pairs:
person = {"name": "Alice", "age": 25}
print(person["name"]) # Output: Alice
These data structures will make your programs more powerful.
Step 7: Error Handling - Avoiding Program Crashes
Mistakes happen! That’s why error handling is essential. Python uses try-except blocks:
try:
num = int(input("Enter a number: "))
print("You entered:", num)
except ValueError:
print("Oops! That’s not a valid number.")
Try running this with both numbers and text to see how Python prevents crashes.
Hurray!!!
Congratulations! You’ve taken your first steps into the world of Python. But this is just the beginning! Challenge yourself by building small projects like a calculator, a to-do list, or even a simple chatbot.
The key to mastering Python is practice. Keep experimenting, keep coding, and most importantly, have fun! Python isn’t just a programming language; it’s your new superpower. By following these steps, you begin your journey towards mastering python programming.
What’s the first Python project you’re excited to build? A simple calculator, a to-do list, or maybe even a fun chatbot? Share your ideas and let’s code together! Happy new month to you all.
Author: David C. Igberi
Interesting!, I followed every step and got them right👌, can't wait to start creating things with this language after mastery, please post more this python language.
Beautiful, but I have been trying for a while now, I still haven't gotten it, it's not that easy o, please throw more light on it, I'm really interested in learning this language.
I have a question though, the step 2 is not quite clear, is there anything we need to do before writing the print("Hello!, World")? I'm confused.
I love the step by step details on how to code.